Add to array only within scope of function
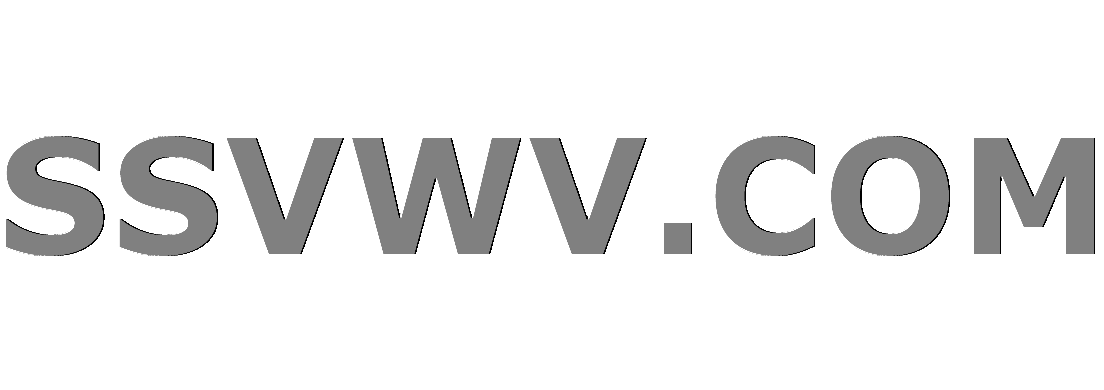
Multi tool use
I'm writing a function that will make a REST API calls which could be either GET
, PUT
, DELETE
, POST
, etc.
I would like to feed this method to the function as a parameter and add it to the options array for that single function call. Is this possible?
Currently I am solving this by creating a separate local
array but would prefer to only use the single options
array.
#!/bin/bash
options=(
--user me:some-token
-H "Accept: application/json"
)
some_func () {
local urn=$1
shift
local func_opts=("${options[@]}" "$@")
printf '%sn' "${func_opts[@]}"
}
# This should return all options including -X GET
some_func /test -X GET
# This should return only the original options
printf '%sn' "${options[@]}"
I could also use a temporary array to store the contents of options
, add the new options, and then reset it before the function ends, but I don't think that is a particularly clean method either.
bash array function
add a comment |
I'm writing a function that will make a REST API calls which could be either GET
, PUT
, DELETE
, POST
, etc.
I would like to feed this method to the function as a parameter and add it to the options array for that single function call. Is this possible?
Currently I am solving this by creating a separate local
array but would prefer to only use the single options
array.
#!/bin/bash
options=(
--user me:some-token
-H "Accept: application/json"
)
some_func () {
local urn=$1
shift
local func_opts=("${options[@]}" "$@")
printf '%sn' "${func_opts[@]}"
}
# This should return all options including -X GET
some_func /test -X GET
# This should return only the original options
printf '%sn' "${options[@]}"
I could also use a temporary array to store the contents of options
, add the new options, and then reset it before the function ends, but I don't think that is a particularly clean method either.
bash array function
add a comment |
I'm writing a function that will make a REST API calls which could be either GET
, PUT
, DELETE
, POST
, etc.
I would like to feed this method to the function as a parameter and add it to the options array for that single function call. Is this possible?
Currently I am solving this by creating a separate local
array but would prefer to only use the single options
array.
#!/bin/bash
options=(
--user me:some-token
-H "Accept: application/json"
)
some_func () {
local urn=$1
shift
local func_opts=("${options[@]}" "$@")
printf '%sn' "${func_opts[@]}"
}
# This should return all options including -X GET
some_func /test -X GET
# This should return only the original options
printf '%sn' "${options[@]}"
I could also use a temporary array to store the contents of options
, add the new options, and then reset it before the function ends, but I don't think that is a particularly clean method either.
bash array function
I'm writing a function that will make a REST API calls which could be either GET
, PUT
, DELETE
, POST
, etc.
I would like to feed this method to the function as a parameter and add it to the options array for that single function call. Is this possible?
Currently I am solving this by creating a separate local
array but would prefer to only use the single options
array.
#!/bin/bash
options=(
--user me:some-token
-H "Accept: application/json"
)
some_func () {
local urn=$1
shift
local func_opts=("${options[@]}" "$@")
printf '%sn' "${func_opts[@]}"
}
# This should return all options including -X GET
some_func /test -X GET
# This should return only the original options
printf '%sn' "${options[@]}"
I could also use a temporary array to store the contents of options
, add the new options, and then reset it before the function ends, but I don't think that is a particularly clean method either.
bash array function
bash array function
asked 7 hours ago


Jesse_bJesse_b
12.6k23067
12.6k23067
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
One option would be to explicitly use a subshell for the function, then override its local copy of the array, knowing that once the subshell exits, the original variable is unchanged:
# a subshell in () instead of the common {}, in order to munge a local copy of "options"
some_func () (
local urn=$1
shift
options+=("$@")
printf '%sn' "${options[@]}"
)
add a comment |
With bash 5.0 and above, you can use the localvar_inherit
option which causes local
to behave like in ash-based shells, that is where local var
makes the variable local without changing its value or attributes:
shopt -s localvar_inherit
options=(
--user me:some-token
-H "Accept: application/json"
)
some_func () {
local urn=$1
shift
local options # make it local, does not change the type nor value
options+=("$@")
printf '%sn' "${options[@]}"
}
some_func /test -X GET
With any version, you can also do:
some_func () {
local urn=$1
shift
eval "$(typeset -p options)" # make a local copy of the outer scope's variable
options+=("$@")
printf '%sn' "${options[@]}"
}
add a comment |
Your Answer
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "106"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2funix.stackexchange.com%2fquestions%2f499553%2fadd-to-array-only-within-scope-of-function%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
One option would be to explicitly use a subshell for the function, then override its local copy of the array, knowing that once the subshell exits, the original variable is unchanged:
# a subshell in () instead of the common {}, in order to munge a local copy of "options"
some_func () (
local urn=$1
shift
options+=("$@")
printf '%sn' "${options[@]}"
)
add a comment |
One option would be to explicitly use a subshell for the function, then override its local copy of the array, knowing that once the subshell exits, the original variable is unchanged:
# a subshell in () instead of the common {}, in order to munge a local copy of "options"
some_func () (
local urn=$1
shift
options+=("$@")
printf '%sn' "${options[@]}"
)
add a comment |
One option would be to explicitly use a subshell for the function, then override its local copy of the array, knowing that once the subshell exits, the original variable is unchanged:
# a subshell in () instead of the common {}, in order to munge a local copy of "options"
some_func () (
local urn=$1
shift
options+=("$@")
printf '%sn' "${options[@]}"
)
One option would be to explicitly use a subshell for the function, then override its local copy of the array, knowing that once the subshell exits, the original variable is unchanged:
# a subshell in () instead of the common {}, in order to munge a local copy of "options"
some_func () (
local urn=$1
shift
options+=("$@")
printf '%sn' "${options[@]}"
)
answered 7 hours ago


Jeff SchallerJeff Schaller
41.2k1056131
41.2k1056131
add a comment |
add a comment |
With bash 5.0 and above, you can use the localvar_inherit
option which causes local
to behave like in ash-based shells, that is where local var
makes the variable local without changing its value or attributes:
shopt -s localvar_inherit
options=(
--user me:some-token
-H "Accept: application/json"
)
some_func () {
local urn=$1
shift
local options # make it local, does not change the type nor value
options+=("$@")
printf '%sn' "${options[@]}"
}
some_func /test -X GET
With any version, you can also do:
some_func () {
local urn=$1
shift
eval "$(typeset -p options)" # make a local copy of the outer scope's variable
options+=("$@")
printf '%sn' "${options[@]}"
}
add a comment |
With bash 5.0 and above, you can use the localvar_inherit
option which causes local
to behave like in ash-based shells, that is where local var
makes the variable local without changing its value or attributes:
shopt -s localvar_inherit
options=(
--user me:some-token
-H "Accept: application/json"
)
some_func () {
local urn=$1
shift
local options # make it local, does not change the type nor value
options+=("$@")
printf '%sn' "${options[@]}"
}
some_func /test -X GET
With any version, you can also do:
some_func () {
local urn=$1
shift
eval "$(typeset -p options)" # make a local copy of the outer scope's variable
options+=("$@")
printf '%sn' "${options[@]}"
}
add a comment |
With bash 5.0 and above, you can use the localvar_inherit
option which causes local
to behave like in ash-based shells, that is where local var
makes the variable local without changing its value or attributes:
shopt -s localvar_inherit
options=(
--user me:some-token
-H "Accept: application/json"
)
some_func () {
local urn=$1
shift
local options # make it local, does not change the type nor value
options+=("$@")
printf '%sn' "${options[@]}"
}
some_func /test -X GET
With any version, you can also do:
some_func () {
local urn=$1
shift
eval "$(typeset -p options)" # make a local copy of the outer scope's variable
options+=("$@")
printf '%sn' "${options[@]}"
}
With bash 5.0 and above, you can use the localvar_inherit
option which causes local
to behave like in ash-based shells, that is where local var
makes the variable local without changing its value or attributes:
shopt -s localvar_inherit
options=(
--user me:some-token
-H "Accept: application/json"
)
some_func () {
local urn=$1
shift
local options # make it local, does not change the type nor value
options+=("$@")
printf '%sn' "${options[@]}"
}
some_func /test -X GET
With any version, you can also do:
some_func () {
local urn=$1
shift
eval "$(typeset -p options)" # make a local copy of the outer scope's variable
options+=("$@")
printf '%sn' "${options[@]}"
}
answered 5 hours ago


Stéphane ChazelasStéphane Chazelas
305k57574928
305k57574928
add a comment |
add a comment |
Thanks for contributing an answer to Unix & Linux Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2funix.stackexchange.com%2fquestions%2f499553%2fadd-to-array-only-within-scope-of-function%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Xq2F,weOGbSrN0g9ZNbzgIa1lGwXWmG3pWkJGDiYYmRbwGo3l8dxKb