How to check if string is entirely made of same substring?
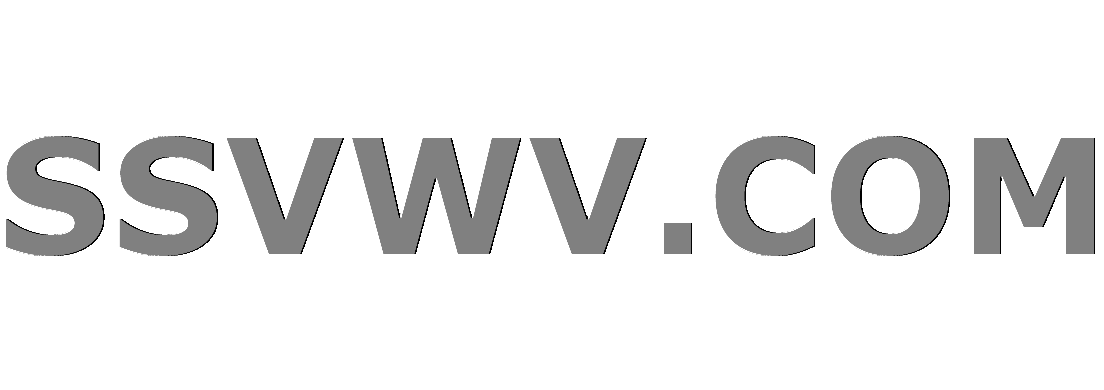
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I have to create a function which takes a string should return true
or false
based on the string. The length of given string is always greater than 1
.
"aa" // true(entirely contains two strings "a")
"aaa" //true(entirely contains three string "a")
"abcabcabc" //true(entirely containas three strings "abc")
"aba" //false(At least there should be two same substrings and nothing more)
"ababa" //false("ab" exists twice but "a" is extra so false)
I have created a function but its
function check(str){
if(!(str.length && str.length - 1)) return false;
let temp = '';
for(let i = 0;i<=str.length/2;i++){
temp += str[i]
//console.log(str.replace(new RegExp(temp,"g"),''))
if(!str.replace(new RegExp(temp,"g"),'')) return true;
}
return false;
}
console.log(check('aa')) //true
console.log(check('aaa')) //true
console.log(check('abcabcabc')) //true
console.log(check('aba')) //false
console.log(check('ababa')) //false
Checking of this is part of real problem. I can't afford a non-efficient solution like this. First of all its looping through half of the string. Second problem is that its using replace()
in each loop which makes it slow.
Can some please suggest be a better solution regarding performance?
javascript algorithm
add a comment |
I have to create a function which takes a string should return true
or false
based on the string. The length of given string is always greater than 1
.
"aa" // true(entirely contains two strings "a")
"aaa" //true(entirely contains three string "a")
"abcabcabc" //true(entirely containas three strings "abc")
"aba" //false(At least there should be two same substrings and nothing more)
"ababa" //false("ab" exists twice but "a" is extra so false)
I have created a function but its
function check(str){
if(!(str.length && str.length - 1)) return false;
let temp = '';
for(let i = 0;i<=str.length/2;i++){
temp += str[i]
//console.log(str.replace(new RegExp(temp,"g"),''))
if(!str.replace(new RegExp(temp,"g"),'')) return true;
}
return false;
}
console.log(check('aa')) //true
console.log(check('aaa')) //true
console.log(check('abcabcabc')) //true
console.log(check('aba')) //false
console.log(check('ababa')) //false
Checking of this is part of real problem. I can't afford a non-efficient solution like this. First of all its looping through half of the string. Second problem is that its using replace()
in each loop which makes it slow.
Can some please suggest be a better solution regarding performance?
javascript algorithm
2
This link may be useful to you. I always find geekforgeeks as a good source for algorithm problems - geeksforgeeks.org/…
– Leron
46 mins ago
I think its a problem of checking whether a common substring occurs throughout uniformly
– Kunal Mukherjee
39 mins ago
add a comment |
I have to create a function which takes a string should return true
or false
based on the string. The length of given string is always greater than 1
.
"aa" // true(entirely contains two strings "a")
"aaa" //true(entirely contains three string "a")
"abcabcabc" //true(entirely containas three strings "abc")
"aba" //false(At least there should be two same substrings and nothing more)
"ababa" //false("ab" exists twice but "a" is extra so false)
I have created a function but its
function check(str){
if(!(str.length && str.length - 1)) return false;
let temp = '';
for(let i = 0;i<=str.length/2;i++){
temp += str[i]
//console.log(str.replace(new RegExp(temp,"g"),''))
if(!str.replace(new RegExp(temp,"g"),'')) return true;
}
return false;
}
console.log(check('aa')) //true
console.log(check('aaa')) //true
console.log(check('abcabcabc')) //true
console.log(check('aba')) //false
console.log(check('ababa')) //false
Checking of this is part of real problem. I can't afford a non-efficient solution like this. First of all its looping through half of the string. Second problem is that its using replace()
in each loop which makes it slow.
Can some please suggest be a better solution regarding performance?
javascript algorithm
I have to create a function which takes a string should return true
or false
based on the string. The length of given string is always greater than 1
.
"aa" // true(entirely contains two strings "a")
"aaa" //true(entirely contains three string "a")
"abcabcabc" //true(entirely containas three strings "abc")
"aba" //false(At least there should be two same substrings and nothing more)
"ababa" //false("ab" exists twice but "a" is extra so false)
I have created a function but its
function check(str){
if(!(str.length && str.length - 1)) return false;
let temp = '';
for(let i = 0;i<=str.length/2;i++){
temp += str[i]
//console.log(str.replace(new RegExp(temp,"g"),''))
if(!str.replace(new RegExp(temp,"g"),'')) return true;
}
return false;
}
console.log(check('aa')) //true
console.log(check('aaa')) //true
console.log(check('abcabcabc')) //true
console.log(check('aba')) //false
console.log(check('ababa')) //false
Checking of this is part of real problem. I can't afford a non-efficient solution like this. First of all its looping through half of the string. Second problem is that its using replace()
in each loop which makes it slow.
Can some please suggest be a better solution regarding performance?
function check(str){
if(!(str.length && str.length - 1)) return false;
let temp = '';
for(let i = 0;i<=str.length/2;i++){
temp += str[i]
//console.log(str.replace(new RegExp(temp,"g"),''))
if(!str.replace(new RegExp(temp,"g"),'')) return true;
}
return false;
}
console.log(check('aa')) //true
console.log(check('aaa')) //true
console.log(check('abcabcabc')) //true
console.log(check('aba')) //false
console.log(check('ababa')) //false
function check(str){
if(!(str.length && str.length - 1)) return false;
let temp = '';
for(let i = 0;i<=str.length/2;i++){
temp += str[i]
//console.log(str.replace(new RegExp(temp,"g"),''))
if(!str.replace(new RegExp(temp,"g"),'')) return true;
}
return false;
}
console.log(check('aa')) //true
console.log(check('aaa')) //true
console.log(check('abcabcabc')) //true
console.log(check('aba')) //false
console.log(check('ababa')) //false
javascript algorithm
javascript algorithm
asked 56 mins ago


Maheer AliMaheer Ali
12.6k1128
12.6k1128
2
This link may be useful to you. I always find geekforgeeks as a good source for algorithm problems - geeksforgeeks.org/…
– Leron
46 mins ago
I think its a problem of checking whether a common substring occurs throughout uniformly
– Kunal Mukherjee
39 mins ago
add a comment |
2
This link may be useful to you. I always find geekforgeeks as a good source for algorithm problems - geeksforgeeks.org/…
– Leron
46 mins ago
I think its a problem of checking whether a common substring occurs throughout uniformly
– Kunal Mukherjee
39 mins ago
2
2
This link may be useful to you. I always find geekforgeeks as a good source for algorithm problems - geeksforgeeks.org/…
– Leron
46 mins ago
This link may be useful to you. I always find geekforgeeks as a good source for algorithm problems - geeksforgeeks.org/…
– Leron
46 mins ago
I think its a problem of checking whether a common substring occurs throughout uniformly
– Kunal Mukherjee
39 mins ago
I think its a problem of checking whether a common substring occurs throughout uniformly
– Kunal Mukherjee
39 mins ago
add a comment |
3 Answers
3
active
oldest
votes
You can do it by capturing group and backreference, just check it's the repetition of first captured value.
function check(str) {
return /^(.+)1+$/.test(str)
}
console.log(check('aa')) //true
console.log(check('aaa')) //true
console.log(check('abcabcabc')) //true
console.log(check('aba')) //false
console.log(check('ababa')) //false
In the above RegExp :
^
and$
stands for start and end anchors to predict the position.
(.+)
captures any pattern and captures the value(exceptn
).
1
is backreference of first captured value and1+
would check for repetition of captured value.
Regex explanation here
Performance difference : https://jsperf.com/reegx-and-loop/1
Can you explain to us what this line is doing return /^(.+)1+$/.test(str)
– Thanveer Shah
47 mins ago
5
Also what is the complexity of this solution? I'm not absolutely sure but it doesn't seem to be much faster than the one the OP has.
– Leron
43 mins ago
@PranavCBalan I'm not good at algorithms, that's why I write in the comments section. However I have several things to mention - the OP already has a working solution so he is asking for one that will give him better performance and you haven't explained how your solution will outperform his. Shorter doesn't mean faster. Also, from the link you gave:If you use normal (TCS:no backreference, concatenation,alternation,Kleene star) regexp and regexp is already compiled then it's O(n).
but as you wrote you are using backreference so is it still O(n)?
– Leron
33 mins ago
@PranavCBalan Its showing both are fastest.
– Maheer Ali
28 mins ago
@MaheerAli : for me, it's showing existing code is44% slower
– Pranav C Balan
27 mins ago
|
show 8 more comments
The fastest algorithmic approach is building Z-function in linear time
C++ implementation for reference (I can't say what JS implementation is good)
vector<int> z_function(string s) {
int n = (int) s.length();
vector<int> z(n);
for (int i = 1, l = 0, r = 0; i < n; ++i) {
if (i <= r)
z[i] = min (r - i + 1, z[i - l]);
while (i + z[i] < n && s[z[i]] == s[i + z[i]])
++z[i];
if (i + z[i] - 1 > r)
l = i, r = i + z[i] - 1;
}
return z;
}
Then you need to check indexes i
that divide n.
If you find such i
that i+z[i]=n
then the string s
can be compressed to the length i
and you can return true
add a comment |
One of the simple idea, replace the string with the substring of "" and it any text exist then it is false ,else it is true.
'ababababa'.replace(/ab/gi,'')
"a" // return false
'abababab'.replace(/ab/gi,'')
""// return true
What aboutabcabcabc
orunicornunicorn
?
– adiga
44 mins ago
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f55823298%2fhow-to-check-if-string-is-entirely-made-of-same-substring%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can do it by capturing group and backreference, just check it's the repetition of first captured value.
function check(str) {
return /^(.+)1+$/.test(str)
}
console.log(check('aa')) //true
console.log(check('aaa')) //true
console.log(check('abcabcabc')) //true
console.log(check('aba')) //false
console.log(check('ababa')) //false
In the above RegExp :
^
and$
stands for start and end anchors to predict the position.
(.+)
captures any pattern and captures the value(exceptn
).
1
is backreference of first captured value and1+
would check for repetition of captured value.
Regex explanation here
Performance difference : https://jsperf.com/reegx-and-loop/1
Can you explain to us what this line is doing return /^(.+)1+$/.test(str)
– Thanveer Shah
47 mins ago
5
Also what is the complexity of this solution? I'm not absolutely sure but it doesn't seem to be much faster than the one the OP has.
– Leron
43 mins ago
@PranavCBalan I'm not good at algorithms, that's why I write in the comments section. However I have several things to mention - the OP already has a working solution so he is asking for one that will give him better performance and you haven't explained how your solution will outperform his. Shorter doesn't mean faster. Also, from the link you gave:If you use normal (TCS:no backreference, concatenation,alternation,Kleene star) regexp and regexp is already compiled then it's O(n).
but as you wrote you are using backreference so is it still O(n)?
– Leron
33 mins ago
@PranavCBalan Its showing both are fastest.
– Maheer Ali
28 mins ago
@MaheerAli : for me, it's showing existing code is44% slower
– Pranav C Balan
27 mins ago
|
show 8 more comments
You can do it by capturing group and backreference, just check it's the repetition of first captured value.
function check(str) {
return /^(.+)1+$/.test(str)
}
console.log(check('aa')) //true
console.log(check('aaa')) //true
console.log(check('abcabcabc')) //true
console.log(check('aba')) //false
console.log(check('ababa')) //false
In the above RegExp :
^
and$
stands for start and end anchors to predict the position.
(.+)
captures any pattern and captures the value(exceptn
).
1
is backreference of first captured value and1+
would check for repetition of captured value.
Regex explanation here
Performance difference : https://jsperf.com/reegx-and-loop/1
Can you explain to us what this line is doing return /^(.+)1+$/.test(str)
– Thanveer Shah
47 mins ago
5
Also what is the complexity of this solution? I'm not absolutely sure but it doesn't seem to be much faster than the one the OP has.
– Leron
43 mins ago
@PranavCBalan I'm not good at algorithms, that's why I write in the comments section. However I have several things to mention - the OP already has a working solution so he is asking for one that will give him better performance and you haven't explained how your solution will outperform his. Shorter doesn't mean faster. Also, from the link you gave:If you use normal (TCS:no backreference, concatenation,alternation,Kleene star) regexp and regexp is already compiled then it's O(n).
but as you wrote you are using backreference so is it still O(n)?
– Leron
33 mins ago
@PranavCBalan Its showing both are fastest.
– Maheer Ali
28 mins ago
@MaheerAli : for me, it's showing existing code is44% slower
– Pranav C Balan
27 mins ago
|
show 8 more comments
You can do it by capturing group and backreference, just check it's the repetition of first captured value.
function check(str) {
return /^(.+)1+$/.test(str)
}
console.log(check('aa')) //true
console.log(check('aaa')) //true
console.log(check('abcabcabc')) //true
console.log(check('aba')) //false
console.log(check('ababa')) //false
In the above RegExp :
^
and$
stands for start and end anchors to predict the position.
(.+)
captures any pattern and captures the value(exceptn
).
1
is backreference of first captured value and1+
would check for repetition of captured value.
Regex explanation here
Performance difference : https://jsperf.com/reegx-and-loop/1
You can do it by capturing group and backreference, just check it's the repetition of first captured value.
function check(str) {
return /^(.+)1+$/.test(str)
}
console.log(check('aa')) //true
console.log(check('aaa')) //true
console.log(check('abcabcabc')) //true
console.log(check('aba')) //false
console.log(check('ababa')) //false
In the above RegExp :
^
and$
stands for start and end anchors to predict the position.
(.+)
captures any pattern and captures the value(exceptn
).
1
is backreference of first captured value and1+
would check for repetition of captured value.
Regex explanation here
Performance difference : https://jsperf.com/reegx-and-loop/1
function check(str) {
return /^(.+)1+$/.test(str)
}
console.log(check('aa')) //true
console.log(check('aaa')) //true
console.log(check('abcabcabc')) //true
console.log(check('aba')) //false
console.log(check('ababa')) //false
function check(str) {
return /^(.+)1+$/.test(str)
}
console.log(check('aa')) //true
console.log(check('aaa')) //true
console.log(check('abcabcabc')) //true
console.log(check('aba')) //false
console.log(check('ababa')) //false
edited 25 mins ago
answered 48 mins ago


Pranav C BalanPranav C Balan
91k1491118
91k1491118
Can you explain to us what this line is doing return /^(.+)1+$/.test(str)
– Thanveer Shah
47 mins ago
5
Also what is the complexity of this solution? I'm not absolutely sure but it doesn't seem to be much faster than the one the OP has.
– Leron
43 mins ago
@PranavCBalan I'm not good at algorithms, that's why I write in the comments section. However I have several things to mention - the OP already has a working solution so he is asking for one that will give him better performance and you haven't explained how your solution will outperform his. Shorter doesn't mean faster. Also, from the link you gave:If you use normal (TCS:no backreference, concatenation,alternation,Kleene star) regexp and regexp is already compiled then it's O(n).
but as you wrote you are using backreference so is it still O(n)?
– Leron
33 mins ago
@PranavCBalan Its showing both are fastest.
– Maheer Ali
28 mins ago
@MaheerAli : for me, it's showing existing code is44% slower
– Pranav C Balan
27 mins ago
|
show 8 more comments
Can you explain to us what this line is doing return /^(.+)1+$/.test(str)
– Thanveer Shah
47 mins ago
5
Also what is the complexity of this solution? I'm not absolutely sure but it doesn't seem to be much faster than the one the OP has.
– Leron
43 mins ago
@PranavCBalan I'm not good at algorithms, that's why I write in the comments section. However I have several things to mention - the OP already has a working solution so he is asking for one that will give him better performance and you haven't explained how your solution will outperform his. Shorter doesn't mean faster. Also, from the link you gave:If you use normal (TCS:no backreference, concatenation,alternation,Kleene star) regexp and regexp is already compiled then it's O(n).
but as you wrote you are using backreference so is it still O(n)?
– Leron
33 mins ago
@PranavCBalan Its showing both are fastest.
– Maheer Ali
28 mins ago
@MaheerAli : for me, it's showing existing code is44% slower
– Pranav C Balan
27 mins ago
Can you explain to us what this line is doing return /^(.+)1+$/.test(str)
– Thanveer Shah
47 mins ago
Can you explain to us what this line is doing return /^(.+)1+$/.test(str)
– Thanveer Shah
47 mins ago
5
5
Also what is the complexity of this solution? I'm not absolutely sure but it doesn't seem to be much faster than the one the OP has.
– Leron
43 mins ago
Also what is the complexity of this solution? I'm not absolutely sure but it doesn't seem to be much faster than the one the OP has.
– Leron
43 mins ago
@PranavCBalan I'm not good at algorithms, that's why I write in the comments section. However I have several things to mention - the OP already has a working solution so he is asking for one that will give him better performance and you haven't explained how your solution will outperform his. Shorter doesn't mean faster. Also, from the link you gave:
If you use normal (TCS:no backreference, concatenation,alternation,Kleene star) regexp and regexp is already compiled then it's O(n).
but as you wrote you are using backreference so is it still O(n)?– Leron
33 mins ago
@PranavCBalan I'm not good at algorithms, that's why I write in the comments section. However I have several things to mention - the OP already has a working solution so he is asking for one that will give him better performance and you haven't explained how your solution will outperform his. Shorter doesn't mean faster. Also, from the link you gave:
If you use normal (TCS:no backreference, concatenation,alternation,Kleene star) regexp and regexp is already compiled then it's O(n).
but as you wrote you are using backreference so is it still O(n)?– Leron
33 mins ago
@PranavCBalan Its showing both are fastest.
– Maheer Ali
28 mins ago
@PranavCBalan Its showing both are fastest.
– Maheer Ali
28 mins ago
@MaheerAli : for me, it's showing existing code is
44% slower
– Pranav C Balan
27 mins ago
@MaheerAli : for me, it's showing existing code is
44% slower
– Pranav C Balan
27 mins ago
|
show 8 more comments
The fastest algorithmic approach is building Z-function in linear time
C++ implementation for reference (I can't say what JS implementation is good)
vector<int> z_function(string s) {
int n = (int) s.length();
vector<int> z(n);
for (int i = 1, l = 0, r = 0; i < n; ++i) {
if (i <= r)
z[i] = min (r - i + 1, z[i - l]);
while (i + z[i] < n && s[z[i]] == s[i + z[i]])
++z[i];
if (i + z[i] - 1 > r)
l = i, r = i + z[i] - 1;
}
return z;
}
Then you need to check indexes i
that divide n.
If you find such i
that i+z[i]=n
then the string s
can be compressed to the length i
and you can return true
add a comment |
The fastest algorithmic approach is building Z-function in linear time
C++ implementation for reference (I can't say what JS implementation is good)
vector<int> z_function(string s) {
int n = (int) s.length();
vector<int> z(n);
for (int i = 1, l = 0, r = 0; i < n; ++i) {
if (i <= r)
z[i] = min (r - i + 1, z[i - l]);
while (i + z[i] < n && s[z[i]] == s[i + z[i]])
++z[i];
if (i + z[i] - 1 > r)
l = i, r = i + z[i] - 1;
}
return z;
}
Then you need to check indexes i
that divide n.
If you find such i
that i+z[i]=n
then the string s
can be compressed to the length i
and you can return true
add a comment |
The fastest algorithmic approach is building Z-function in linear time
C++ implementation for reference (I can't say what JS implementation is good)
vector<int> z_function(string s) {
int n = (int) s.length();
vector<int> z(n);
for (int i = 1, l = 0, r = 0; i < n; ++i) {
if (i <= r)
z[i] = min (r - i + 1, z[i - l]);
while (i + z[i] < n && s[z[i]] == s[i + z[i]])
++z[i];
if (i + z[i] - 1 > r)
l = i, r = i + z[i] - 1;
}
return z;
}
Then you need to check indexes i
that divide n.
If you find such i
that i+z[i]=n
then the string s
can be compressed to the length i
and you can return true
The fastest algorithmic approach is building Z-function in linear time
C++ implementation for reference (I can't say what JS implementation is good)
vector<int> z_function(string s) {
int n = (int) s.length();
vector<int> z(n);
for (int i = 1, l = 0, r = 0; i < n; ++i) {
if (i <= r)
z[i] = min (r - i + 1, z[i - l]);
while (i + z[i] < n && s[z[i]] == s[i + z[i]])
++z[i];
if (i + z[i] - 1 > r)
l = i, r = i + z[i] - 1;
}
return z;
}
Then you need to check indexes i
that divide n.
If you find such i
that i+z[i]=n
then the string s
can be compressed to the length i
and you can return true
answered 23 mins ago
MBoMBo
50.6k23152
50.6k23152
add a comment |
add a comment |
One of the simple idea, replace the string with the substring of "" and it any text exist then it is false ,else it is true.
'ababababa'.replace(/ab/gi,'')
"a" // return false
'abababab'.replace(/ab/gi,'')
""// return true
What aboutabcabcabc
orunicornunicorn
?
– adiga
44 mins ago
add a comment |
One of the simple idea, replace the string with the substring of "" and it any text exist then it is false ,else it is true.
'ababababa'.replace(/ab/gi,'')
"a" // return false
'abababab'.replace(/ab/gi,'')
""// return true
What aboutabcabcabc
orunicornunicorn
?
– adiga
44 mins ago
add a comment |
One of the simple idea, replace the string with the substring of "" and it any text exist then it is false ,else it is true.
'ababababa'.replace(/ab/gi,'')
"a" // return false
'abababab'.replace(/ab/gi,'')
""// return true
One of the simple idea, replace the string with the substring of "" and it any text exist then it is false ,else it is true.
'ababababa'.replace(/ab/gi,'')
"a" // return false
'abababab'.replace(/ab/gi,'')
""// return true
'ababababa'.replace(/ab/gi,'')
"a" // return false
'abababab'.replace(/ab/gi,'')
""// return true
'ababababa'.replace(/ab/gi,'')
"a" // return false
'abababab'.replace(/ab/gi,'')
""// return true
answered 46 mins ago


Vinod kumar GVinod kumar G
435412
435412
What aboutabcabcabc
orunicornunicorn
?
– adiga
44 mins ago
add a comment |
What aboutabcabcabc
orunicornunicorn
?
– adiga
44 mins ago
What about
abcabcabc
or unicornunicorn
?– adiga
44 mins ago
What about
abcabcabc
or unicornunicorn
?– adiga
44 mins ago
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f55823298%2fhow-to-check-if-string-is-entirely-made-of-same-substring%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
KeR,5ChKkjptCLJNTwdAfnplBHM7,GFziyE k5I Z
2
This link may be useful to you. I always find geekforgeeks as a good source for algorithm problems - geeksforgeeks.org/…
– Leron
46 mins ago
I think its a problem of checking whether a common substring occurs throughout uniformly
– Kunal Mukherjee
39 mins ago